JavaScript explained by examples. Widgets and scripts
It is easier to learn a language with interative examples. This tutorial, that is also a reference manual, offers interactive demos for each element of JavaScript.
Summary of the JavaScript programming
language
If you know C, Java or PHP, moving to JavaScript is very easy. This page
is a reference to help in use of our examples and demos.
JavaScript and C: Inheritance and differences
Variables and constants
Variables are dynamic in JavaScript.
- Number
- String
- Array
- Date
- Boolean
- Scope of variables.
- Can we pass variables by reference in JavaScript?
Array
They are dynamic and have attributes and methods.
- Associative array.
- For each.
- Pitfalls in arrays.
- New methods of Array.
- Comprehension array. How to modify the content of an array of filter it with just one command.
Functions
Defining and calling functions.
- Built-in functions.
- The eval function. This built-in function allows to add variables and functions dynamically.
- The bind method. To solve this issues in callbacks.
Control structures
Loops and conditional expressions.
Regular expression
Processing texts with matching rules.
- Div and mouse. To know the position of the mouse inside a div, what properties?
- Optical illusion. Demonstration: How to change an image when the mouse is pressed down on it.
Objects
In JavaScript, they are dynamic, the structure can grow during a processing.
- This and new, a close relationship. How a function becomes an object.
- Copy, avatar and double. Assigning an object to a variable only adds a reference to the same object. How to make an independent copy?
- Class and inheritance in common JavaScript. It is quite possible to define a constructor and inherit from another class without waiting ES6.
JavaScript and PHP
Sharing variables between PHP and Web pages. Adding variables to that
of a form.
Asynchronous JavaScript
How to make JavaScript asynchronous code like that of Analytics from Google?
New features in ECMAScript
And tests of implementation.
- Promise
Running a code only when the result of a processing is obtained. - Async/Await
Examples of use. - Import and module made simple
How to import JS modules in the easiest way possible.
- JavaScript without callback
One can avoid the spaghetti code with named functions or promises, particularly when asynchronous functions are chained. - Converting a callback to a promise
To turn synchronous an asynchronous function, with await. - Proxy
A code simplification tool, proxy allows to separate the bulk of the application of the accessory. - Map and object: converting nested objects
Conversions between Map and object when they embed other Map and objects.
JavaScript cheat sheet
The essential in one page.
Forms
Creating and using a form.
Principles and form objects in HTML 4 and HTML 5. Adding a button or an image used
as button.
List of all form objects in HTML.
Sending and receiving data depending on form objects
Transmitting data to a web page or a script on the server.
- How to pass data to another HTML page. How to pass parameters to a Web page? This is accomplished by a form and a simple script that extracts values from the parameter string.
- GET or POST, what method to choose?
- Frames & forms. How to pass form data between frames of a frameset.
Checkbox, how to pass the state to another page
Select
Displaying a list of items to select.
Table and query with JSON
Storing a table in a JSON file and accessing its content with a SQL-style query.
HTML Objects
Window
Creating dynamically a window in JavaScript.
- Location. Accessing element of the URL of the page.
- History. Interface to the browser history.
- Dialogs. Interacting with the user, with alert, confirm, prompt.
- Opening a window in JavaScript.
- setTimeout and setInterval. Delay before execution.
Navigator
An object to identify the browser of the user.
Image Map
Links in images.
- Dynamic images. Replacing an image.
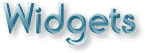
Lightbox without framework
To build a lightbox and display text or images in a box, only two CSS descriptors
are required. And some JavaScript code is added to dynamically change the
contents of the box.
Tab Panel without Framework
A simple Tab Panel for a website or an application in CSS, and to insert another page in the same page with a few lines of JavaScript.
- Tab Panel for HTML application. When the content is already in the page.
- Tab Panel with frames. Second part of the article.
Button switching
How to create a two-states button or textual command.
Scoring article
How to implement a system that allows readers to click on an symbol to
give an article a score.
Mysterious picture
Replace with a fade effect a text by a picture, so that the picture is displayed only when needed.
Navigation bar
Accessing the pages of an article splitted into several parts.
Bookmarklet tutorial
Adding functions to a webpage with just a link in the page on in the bookmarks. Several examples.
- Bookmarklets for search
Advanced search function into links. - Bookmarklets: informations on the page
Know anything about a webpage.